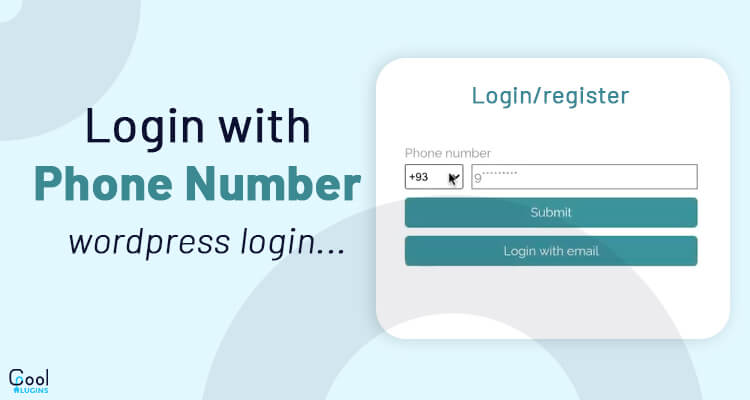
Users create a Login form to Login with Phone Number in WordPress on the front-end while creating their WordPress website. It simply means allowing your users to login with their phone numbers or using their email. Which means you are providing users with options to enter their Phone/Email/Username and a password.
This article does not include a tutorial to show login with OTP rather I am including another option of phone number in addition with email and username. It makes it easier for the user to select either of these options that is suitable for them. The most common example is E-commerce websites like Amazon. It lets the users login with their phone number or email.
Create a login form:
Now I will create a simple login form. On the Submit button, I will write a code in the background that checks the credentials entered by the user for all the options mentioned above(Phone/Email/Username) and a password.
If the user wants to add a phone number on a login form it should be inserted in the ‘wp_usermeta’ table. This is done while the user is registering with his details. The following code shows how to add a user’s phone number.
<?php
$user_id = 1;
$phone_number = 9999999999;
add_user_meta( $user_id, ‘user_phone’, $phone_number);
When you have meta_key ‘user_phone’ and its value stored in the database, you are ready to access the login with phone number option in a login form. The following code shows the login form on your login page.
<?php
$return = log_the_user_in();
if( is_wp_error( $return ) ) {
echo $return->get_error_message();
}
?>
<form method=”post”>
<p><input type=”text” name=”user_login” placeholder=”Username, email or mobile” required /></p>
<p><input type=”password” name=”user_password” placeholder=”Password” required /></p>
<input type=”hidden” name=”login_nonce” value=”<?php echo wp_create_nonce(‘login_nonce’); ?>” />
<input type=”submit” name=”login_the_user” value=”Submit” />
</form>
The above code basically checks for errors and prints them. To handle errors we need to write another code. So we will write code and add nonce which is needed to process the form. It is highly regarded in WordPress.
Login with Phone Number in WordPress
When a user clicks the submit button after filling all his credentials, it is then verified against the data present in the database. If the credentials are correct according to the database, the user will get logged in. If the credentials are incorrect, errors are logged with WP_Error class.
Write the following code in functions.php file. It will process the login form.
<?php
add_action( ‘init’, ‘log_the_user_in’ );
function log_the_user_in() {
if ( isset( $_POST[‘login_the_user’] ) && wp_verify_nonce( $_REQUEST[‘login_nonce’], ‘login_nonce’ ) ) {
if ( ! empty( $_POST[‘user_login’] ) && ! empty( $_POST[‘user_password’] ) ) {
if ( is_email( $_POST[‘user_login’] ) ) {
// check user by email
$user = get_user_by( ’email’, $_POST[‘user_login’] );
} elseif ( is_numeric( $_POST[‘user_login’] ) ) {
// check user by phone number
global $wpdb;
$tbl_usermeta = $wpdb->prefix.’usermeta’;
$user_id = $wpdb->get_var( $wpdb->prepare( “SELECT user_id FROM $tbl_usermeta WHERE meta_key=%s AND meta_value=%s”, ‘user_phone’, $_POST[‘user_login’] ) );
$user = get_user_by( ‘ID’, $user_id );
} else {
// check user by username
$user = get_user_by( ‘login’, $_POST[‘user_login’] );
}
if ( ! $user ) {
return new WP_Error(‘wrong_credentials’, ‘Invalid credentials.’);
}
// check the user’s login with their password.
if ( ! wp_check_password( $_POST[‘user_password’], $user->user_pass, $user->ID ) ) {
return new WP_Error(‘wrong_credentials’, ‘Invalid credentials.’);
}
wp_clear_auth_cookie();
wp_set_current_user($user->ID);
wp_set_auth_cookie($user->ID);
wp_redirect(get_bloginfo(‘url’));
exit;
} else {
return new WP_Error(’empty’, ‘Both fields are required.’);
}
}
}
- At first the nonce is verified to protect our code from misuse, malicious codes and CSRF attacks.
- After that is_email() or is_numeric() checks if the entered email or phone number is correct or not.
- If the entered value is not either of these, then it goes for username.
- If the credentials are correct, the user gets logged in and redirected to the home page or dashboard. Users can be redirected to another page as per the flow.
I hope this article helps you to understand login with a phone number in WordPress.